Codebox Software
Video Barcode Script
Published:
This shell script extracts still frames from video files, and uses them to generate Video Barcode images like these:
Star Wars
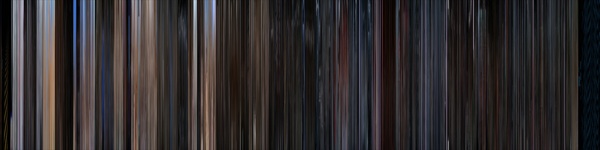
The Empire Strikes Back
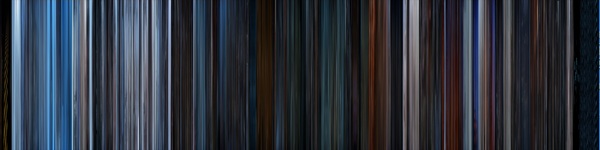
Return of the Jedi
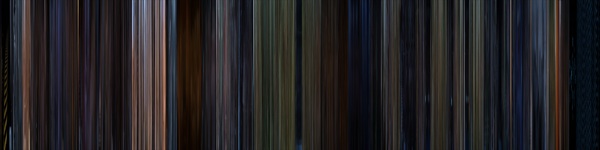
The script should work on Linux and Mac OSX, and has dependencies on ffmpeg and ImageMagick. It is based on an idea by BenoƮt Romito but uses a different technique to create the images, explained below. The source code for this script is shown below, and is also available on GitHub
#!/bin/bash # Set things up... INFILE=$1 if [[ -z "$INFILE" || ! -f $INFILE ]]; then echo Please supply the location of a video file exit 1 fi OUTFILE=$INFILE.jpg STEP=1 CURR=1 TMPDIR=./tmp if [ ! -d $TMPDIR ]; then mkdir $TMPDIR fi # Find the duration of the video, in seconds LEN_TXT=`ffmpeg -i $INFILE 2>&1|grep Duration|cut -f4 -d' '|cut -f1 -d'.'| sed 's/:0/:/g' | sed 's/:/ \\* 3600 + /'|sed 's/:/ \\* 60 + /'` let "LEN=$LEN_TXT" echo "Duration=$LEN seconds" # Ctrl-C to stop function stopnow(){ echo Processing halted, re-execute script with the same file to resume exit 2 } trap stopnow 2 FILENAME=`basename $INFILE` # Make the thumbnail images, and squash them into vertical bars while [ $CURR -le $LEN ] do PADDED_NUM=`printf "%05d" $CURR` THUMBFILE=$TMPDIR/$FILENAME.$PADDED_NUM.jpg BARFILE=$TMPDIR/$FILENAME.bar$PADDED_NUM.jpg if [ ! -f $BARFILE ]; then echo Processing $CURR of $LEN ffmpeg -ss $CURR -i $INFILE -vcodec mjpeg -vframes 1 -y -an -f rawvideo -s 320x240 $THUMBFILE >/dev/null 2>&1 convert $THUMBFILE -resize 1x400\! $BARFILE >/dev/null 2>&1 fi let CURR+=STEP done # Stitch the vertical bars together to make the barcode convert $TMPDIR/$FILENAME.bar* +append $OUTFILE echo Finished.
How it works
The script requires a single command-line argument, which should be the location of the video file for which the barcode is to be generated, for example:makebarcode.sh starwars.avi
The script extracts still frames from the video at one-second intervals, and saves them as JPEG image files (to change the interval between stills, and therefore the length of the barcode, adjust the STEP variable). Each image is then squashed to be only 1 pixel wide, and 400 pixels high, and these squashed images are stitched together horizontally to form a barcode.